CBSE Python
CS-IT-IP-AI and Data Science Notes, QNA for Class 9 to 12
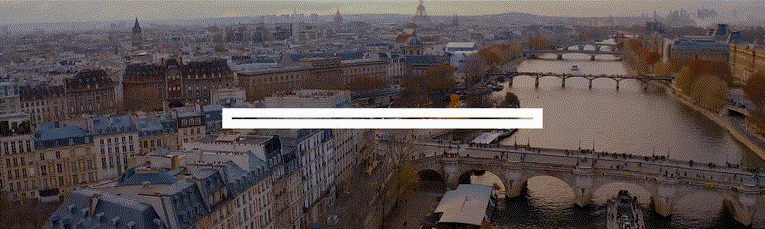
150+ MCQ Revision Tour of python class 12
Unit -1: computational thinking and programming-2, topic: revision of python topics covered in class xi.
1. Which of the following is a valid identifier:
c) Same-type
Show Answer
2. Which of the following is a relational operator:
3. Which of the following is a logical operator:
4. Identify the membership operator from the following:
c) both i & ii
5. Which one is a arithmetic operator:
d) Only ii
6. What will be the correct output of the statement : >>>4//3.0
d) None of the above
7. What will be the correct output of the statement : >>> 4+2**2*10
8. Give the output of the following code:
>>> a,b=4,2
>>> a+b**2*10
9. Give the output of the following code:
>>> a,b = 4,2.5
>>> a-b//2**2
10. Give the output of the following code:
>>>a,b,c=1,2,3
>>> a//b**c+a-c*a
11. If a=1,b=2 and c= 3 then which statement will give the output as : 2.0 from the following:
a) >>>a%b%c+1
b) >>>a%b%c+1.0
c) >>>a%b%c
b) >>>a%b%c+1.0
12. Which statement will give the output as : True from the following :
a) >>>not -5
b) >>>not 5
c) >>>not 0
d) >>>not(5-1)
13. Give the output of the following code:
>>>7*(8/(5//2))
14. Give the output of the following code:
>>>import math
>>> math.ceil(1.03)+math.floor(1.03)
15.What will be the output of the following code:
>>>math.fabs(-5.03)
16.Single line comments in python begin with……………….. symbol.
17.Which of the following are the fundamental building block of a python program.
a) Identifier
b) Constant
c) Punctuators
18.The input() function always returns a value of ……………..type.
19.……….. function is used to determine the data type of a variable.
a) type( )
c) print( )
20.The smallest individual unit in a program is known as a……………
c) punctuator
d) identifier
FLOW OF EXECUTION
#Decision making statements in python Statement
21. Which of the following is not a decision making statement
a) if..else statement
b) for statement
c) if-elif statement
d) if statement
22. …………loop is the best choice when the number of iterations are known.
b) do-while
d) None of these
23. How many times will the following code be executed.
while a>0:
print(“Bye”)
c) Infinite
24. What abandons the current iteration of the loop
a) continue
c) infinite
25. Find the output of the following python program
for i in range(1,15,4):
print(i, end=’,’)
c) 1,5,10,14
d) 1,5,9,13
26. …………loop is the best when the number of iterations are not known.
27.In the nested loop ……………..loop must be terminated before the outer loop.
b) enclosing
28. …………..statement is an empty statement in python.
c) continue
29. How many times will the following code be executed
for i in range(1,15,5):
print(i,end=’,’)
d) infinite
30. Symbol used to end the if statement:
a) Semicolon(;)
b) Hyphen(-)
c) Underscore( _ )
d) colon(:)
String Methods and Built-in functions:
31. Which of the following is not a python legal string operation.
a) ‘abc’+’aba’
c) ‘abc’+3
d) ‘abc’.lower()
32. Which of the following is not a valid string operation.
b) concatenation
c) Repetition
33. Which of the following is a mutable type.
34. What will be the output of the following code
str1=”I love Python”
strlen=len(str1)+5
print(strlen)
35. Which method removes all the leading whitespaces from the left of the string.
b) remove()
c) lstrip()
d) rstrip()
36. It returns True if the string contains only whitespace characters, otherwise returns False.
a) isspace()
c) islower()
d) isupper()
37. It converts uppercase letter to lowercase and vice versa of the given string.
a) lstrip()
b) swapcase()
c) istitle()
38.What will be the output of the following code.
Str=’Hello World! Hello Hello’
Str.count(‘Hello’,12,25)
39.What will be the output of the following code.
Str=”123456”
print(Str.isdigit())
40.What will be the output of the following code.
Str=”python 38”
print(Str.isalnum())
41.What will be the output of the following code.
Str=”pyThOn”
print(Str.swapcase())
42.What will be the output of the following code.
Str=”Computers”
print(Str.rstrip(“rs”))
a) Computer
b) Computers
c) Compute
43.What will be the output of the following code.
Str=”This is Meera\’ pen”
44.How many times is the word ‘Python’ printed in the following statement.
s = ”I love Python”
for ch in s[3:8]:
print(‘Python’)
a) 11 times
d) 5 times
45.Which of the following is the correct syntax of string slicing:
a) str_name[start:end]
b) str_name[start:step]
c) str_name[step:end]
d) str_name[step:start]
46.What will be the output of the following code?
A=”Virtual Reality”
print(A.replace(‘Virtual’,’Augmented’))
a) Virtual Augmented
b) Reality Augmented
c) Augmented Virtual
d) Augmented Reality
47.What will be the output of the following code?
print(“ComputerScience”.split(“er”,2))
a) [“Computer”,”Science”]
b) [“Comput”,”Science”]
c) [“Comput”,”erScience”]
d) [“Comput”,”er”,”Science”]
48.Following set of commands are executed in shell, what will be the output?
>>>str=”hello”
>>>str[:2]
49.………..function will always return tuple of 3 elements.
c) partition()
50.What is the correct python code to display the last four characters of “Digital India”
a) str[-4:]
c) str[*str]
d) str[/4:]
51. Given the list L=[11,22,33,44,55], write the output of print(L[: :-1]).
a) [1,2,3,4,5]
b) [22,33,44,55]
c) [55,44,33,22,11]
d) Error in code
52. Which of the following can add an element at any index in the list?
a) insert( )
b) append( )
c) extend()
d) all of these
53. Which of the following function will return a list containing all the words of the given string?
54.Which of the following statements are True.
i) [1,2,3,4]>[4,5,6]
ii) [1,2,3,4]<[1,5,2,3]
iii) [1,2,3,4]>[1,2,0,3]
iv) [1,2,3,4]<[1,2,3,2]
b) i,iii,iv
c) i,ii,iii
55. If l1=[20,30] l2=[20,30] l3=[‘20’,’30’] l4=[20.0,30.0] then which of the following statements will not return ‘False’:
i) >>>l1==l2
ii) >>>l4>l1
iii) >>>l1>l2
iv) >>> l2==l2
b) i,ii,iii
c) i,iii,iv
56.>>>l1=[10,20,30,40,50]
>>>l2=l1[1:4]
What will be the elements of list l2:
a) [10,30,50]
b) [20,30,40,50]
c) [10,20,30]
d) [20,30,40]
57.>>>l=[‘red’,’blue’]
>>>l = l + ‘yellow’
What will be the elements of list l:
a) [‘red’,’blue’,’yellow’]
b) [‘red’,’yellow’]
c) [‘red’,’blue’,’yellow’]
58.What will be the output of the following code:
>>>l=[1,2,3,4]
>>>m=[5,6,7,8]
>>>n=m+l
>>>print(n)
a) [1,2,3,5,6,7,8]
b) [1,2,3,4,5,6,7,8]
c) [1,2,3,4][5,6,7,8]
59.What will be the output of the following code:
>>>m=l*2
>>>n=m*2
a) [1,2,3,4,1,2,3,4,1,2,3,4]
b) [1,2,3,4,1,2,3,4,1,2,3,4,1,2,3,4]
c) [1,2,3,4][4,5,6,7]
d) [1,2,3,4]
60.Match the columns: if
>>l=list(‘computer’)
a) 1-b,2-d,3-a,4-c
b) 1-c,2-b,3-a,4-d
c) 1-b,2-d,3-c,4-a
d) 1-d,2-a,3-c,4-b
61. If a list is created as
>>>l=[1,2,3,’a’,[‘apple’,’green’],5,6,7,[‘red’,’orange’]] then what will be the output of the following statements:
>>>l[4][1]
b) ‘green’
d) ‘orange’
62.>>>l[8][0][2]
63.>>>l[-1]
a) [‘apple’,’green’]
b) [‘red’,’orange’]
c) [‘red’ ]
d) [’orange’]
64.>>>len(l)
65.What will be the output of the following code:
>>>l1=[1,2,3]
>>>l1.append([5,6])
>>>l1
a) [1,2,3,5,6]
b) [1,2,3,[5,6]]
d) [1,2,3,[5,6]]
66.What will be the output of the following code:
>>>l2=[5,6]
>>>l1.extend(l2)
a) [5,6,1,2,3]
b) [1,2,3,5,6]
d) [1,2,3,6]
67. What will be the output of the following code:
>>>l1.insert(2,25)
a) [1,2,3,25]
b) [1,25,2,3]
c) [1,2,25,3]
d) [25,1,2,3,6]
68. >>>l1=[10,20,30,40,50,60,10,20,10]
>>>l1.count(‘10’)
69.Which operators can be used with list?
c) both (i)&(ii)
d) Arithmetic operator only
70.Which of the following function will return the first occurrence of the specified element in a list.
d) sorted()
MCQ Tuples and Dictionary:
71. Which of the statement(s) is/are correct.
a) Python dictionary is an ordered collection of items.
b) Python dictionary is a mapping of unique keys to values
c) Dictionary is mutable.
d) All of these.
72. ………function is used to convert a sequence data type into tuple.
73. It tup=(20,30,40,50), which of the following is incorrect
a) print(tup[3])
b) tup[2]=55
c) print(max(tup))
d) print(len(tup))
74. Consider two tuples given below:
>>>tup1=(1,2,4,3)
>>>tup2=(1,2,3,4)
What will the following statement print(tup1<tup2)
75. Which function returns the number of elements in the tuple
d) count( )
76. Which function is used to return a value for the given key.
77.Keys of the dictionary must be
c) can be similar or unique
d) All of these
78. Which of the following is correct to insert a single element in a tuple .
79.Which of the following will delete key-value pair for key=’red’ form a dictionary D1
a) Delete D1(“red”)
b) del. D1(“red”)
c) del D1[“red”]
80.Which function is used to remove all items form a particular dictionary.
a) clear( )
81.In dictionary the elements are accessed through
82.Which function will return key-value pairs of the dictionary
b) values( )
c) items( )
83.Elements in a tuple can be of ………….type.
a) Dissimilar
c) both i & ii
84.To create a dictionary , key-value pairs are separated by…………….
85.Which of the following statements are not correct:
i) An element in a dictionary is a combination of key-value pair
ii) A tuple is a mutable data type
iii) We can repeat a key in dictionary
iv) clear( ) function is used to deleted the dictionary.
a) i,ii,iii
b) ii,iii,iv
c) ii,iii,i
d) i,ii,iii,iv
86.Which of the following statements are correct:
i) Lists can be used as keys in a dictionary
ii) A tuple cannot store list as an element
iii) We can use extend() function with tuple.
iv) We cannot delete a dictionary once created.
b) ii,iii,iv
87.Like lists, dictionaries are……………..which mean they can be changed.
a) Mutable
b) immutable
c) variable
88.To create an empty dictionary , we use
d) d= < >
89.To create dictionary with no items , we use
b) dict( )
90.What will be the output
>>>d1={‘rohit’:56,”Raina”:99}
>>>print(“Raina” in d1)
c) No output
91. Rahul has created the a tuple containing some numbers as
>>>t=(10,20,30,40)
now He want to add a new element 60 in the tuple, which statement he should use out of the given four.
a) >>>t+(60)
b) >>>t + 60
c) >>>t + (60,)
d) >>>t + (‘60’)
92. Rahul wants to delete all the elements from the tuple, which statement he should use
a) >>>del t
b) >>>t.clear()
c) >>>t.remove()
d) >>>None of these
93.Rahul wants to display the last element of the tuple, which statement he should use
a) >>> t.display()
b) >>>t.pop()
c) >>>t[-1]
d) >>>t.last()
94.Rahul wants to add a new tuple t1 to the tuple t, which statement he should use
a) >>>t+t1
b) >>>t.add(t1)
c) >>>t*t1
95. Rahul has issued a statement after that the tuple t is replace with empty tuple, identify the statement he had issued out of the following:
a) >>> del t
b) >>>t= tuple()
c) >>>t=Tuple()
d) >>>delete t
96. Rahul wants to count that how many times the number 10 has come:
a) >>>t.count(10)
b) >>>t[10]
c) >>>count.t(10)
97. Rahul want to know that how many elements are there in the tuple t, which statement he should use out of the given four
a) >>>t.count()
b) >>>len(t)
c) >>>count(t)
d) >>>t.sum()
98.>>>t=(1,2,3,4)
Write the statement should be used to print the first three elements 3 times
a) >>>print(t*3)
b) >>>t*3
c) >>>t[:3]*3
d) >>>t+t
99. Match the output with the statement given in column A with Column B.
a) 1-b,2-c,3-d,4-a
b) 1-a,2-c,3-d,4-b
c) 1-c,2-d,3-a,4-a
d) 1-d,2-a,3-b,4-c
100. Write the output of the following code:
>>>d={‘name’:’rohan’,’dob’:’2002-03-11’,’Marks’:’98’}
>>>d1={‘name’:‘raj’)
>>>d1=d.copy()
>>>print(“d1 :”d1)
a) d1 : {‘name’: ‘rohan’, ‘dob’: ‘2002-03-11’, ‘Marks’: ’98’}
b) d1 = {‘name’: ‘rohan’, ‘dob’: ‘2002-03-11’, ‘Marks’: ’98’}
c) {‘name’: ‘rohan’, ‘dob’: ‘2002-03-11’, ‘Marks’: ’98’}
d) (d1 : {‘name’: ‘rohan’, ‘dob’: ‘2002-03-11’, ‘Marks’: ’98’})
Related Post
Class 12 computer science board question paper 2024 with answer key, python function arguments and parameters notes class 12 (positional, keyword, default), class 12 computer science notes topic wise.

CS-IP-Learning-Hub
Important Questions and Notes
Best 400+ MCQ on Python Revision Tour Class 12

Python Revision Tour Class 12 Topic Wise MCQ
1. 140+ MCQ on Introduction to Python
2. 120 MCQ on String in Python
3. 100+ MCQ on List in Python
4. 50+ MCQ on Tuple in Python
5. 100+ MCQ on Flow of Control in Python
6. 60+ MCQ on Dictionary in Python
Important Links
100 practice questions on python fundamentals, 120+ mysql practice questions, 90+ practice questions on list, 50+ output based practice questions, 100 practice questions on string, 70 practice questions on loops, 120 practice questions of computer network in python, 70 practice questions on if-else, 40 practice questions on data structure, python functions mcq class 12 cs.
Computer Science Syllabus 2021-2022 .
Informatics Practices Syllabus 2021-2022
Class 12 Computer Science Chapter wise MCQ
Leave a Reply Cancel reply

THE PATH TO SUCCESS IN EXAM...
Class 12 Computer Science MCQs Python Revision Tour Set – 2
Class 12 computer science 083.
- Class 12 Computer Science MCQs Python Revision Tour Set – 2 by mycstutorial
- Class 12 Computer Science MCQs Python Revision Tour Set – 1 by mycstutorial
Python Revision Tour Set 2
Multiple Choice Questions (MCQs)
21. Is Python case sensitive when dealing with identifiers?
c) Machine dependent
d) None of these
Answer : a) Yes
22. Which of the following is an invalid variable?
a) name_of_student
b) 2_name_of_student
c) student_name_2
Answer : b) 2_name_of_student
23. Which of the following is not a keyboar?
b) nonlocal
Answer : a) eval
24. Which of the following cannot be a variable?
c) __init__
Answer : a) in
25. Which of these is not a a core data type?
c) dictionary
Answer : a) class
26. How do you write p t in Python as an expression?
Answer : b) p**t
27. How you will write the formula of compound interest in Python?
a) p * 1 + r/100 ** t
b) p * 1 + r/100 ** t – p
c) p * (1 + r/100)**t – p
Answer : c) p * 1 + r/100 ** t – p
28. Which of the following is an invalid statement in Python?
a) a=10,500
b) a,b = 10,500
c) a = b = 10
d) a b = 10 500
Answer : d) a b = 10 500
29. Which value type does print() return?
Answer : d) None
30. Which value type does input() return?
Answer : a) String
31. Which of the following is not a valid keyword?
Answer : c) final
32. Which of the following is not a valid keyword?
Answer : b) delete
33. In Python, String can be written in ______ ways?
Answer : b) 2
34. In Python, String can be ___________ .
a) Single line strings
b) Multi-line strings
c) Both (a) and (b)
Answer : c) Both (a) and (b)
35. In Python, String (i.e. sequence of characters) can be surrounded by ___________ .
a) Single quote ‘ ‘
b) Double quote ” “
c) Triple quote ”’ ”’ or “”” “””
d) All of these
Answer : d) All of these
36. Which of the following is a valid multi line string?
a) name = “mycs \
tutorial.in”
b) name =””” mycs
tutorial.in”””
c) name = ”’ mycs
tutorial.in”’
More MCQ’s will be uploaded by 13-12-2021 11:00 pm
Class 12 computer science multiple choice questions (mcq).
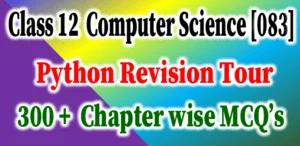
Related Posts
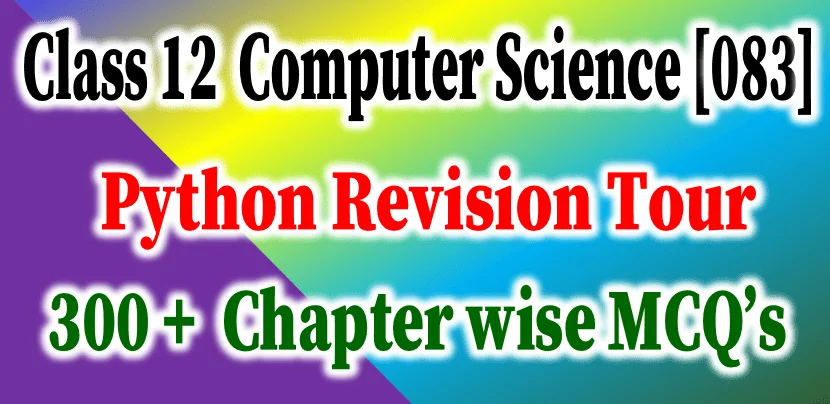
Class 12 Computer Science MCQs Python Revision Tour Set – 1
Leave a comment cancel reply.
You must be logged in to post a comment.
You cannot copy content of this page

Python Revision Tour II
Class 12 - computer science with python sumita arora, multiple choice questions.
The numbered position of a letter in a string is called ...............
- integer position
Reason — The index is the numbered position of a letter in the string.
The operator ............... tells if an element is present in a sequence or not.
Reason — in is membership operator which returns True if a character or a substring exists in the given string else returns False.
The keys of a dictionary must be of ............... types.
- any of these
Reason — Dictionaries are indexed by keys and its keys must be of any immutable type.
Following set of commands is executed in shell, what will be the output?
Reason — str[:2] here slicing operation begins from index 0 and ends at index 1. Hence the output will be 'he'.
What data type is the object below ? L = [1, 23, 'hello', 1]
Reason — A list can store a sequence of values belonging to any data type and they are depicted through square brackets.
What data type is the object below ? L = 1, 23, 'hello', 1
Reason — For creating a tuple, enclosing the elements inside parentheses is optional. Even if parentheses are omitted as shown here, still this statement will create a tuple.
To store values in terms of key and value, what core data type does Python provide ?
Reason — Dictionaries are mutable with elements in the form of a key:value pair that associate keys to values.
What is the value of the following expression ? 3 + 3.00, 3**3.0
(6.0, 27.0)
- (6.0, 9.00)
- [6.0, 27.0]
Reason — The value of expression is in round brackets because it is tuple. 3 + 3.00 = 6.0 3**3.0 = 3 x 3 x 3 = 27.0
List AL is defined as follows : AL = [1, 2, 3, 4, 5] Which of the following statements removes the middle element 3 from it so that the list AL equals [1, 2, 4, 5] ?
- AL[2:3] = []
- AL[2:2] = []
- AL.remove(3)
del AL[2] AL[2:3] = [] AL.remove(3)
Reason — del AL[2] — The del keyword deletes the element from the list AL from index 2. AL[2:3] = [] — The slicing of the list AL[2:3] begins at index 2 and ends at index 2. Therefore, the element at index 2 will be replaced by an empty list []. AL.remove(3) — The remove() function removes an element from the list AL from index 3."
Question 10
Which two lines of code are valid strings in Python ?
- This is a string
- 'This is a string'
- (This is a string)
- "This is a string"
'This is a string' "This is a string"
Reason — Strings are enclosed within single or double quotes.
Question 11
You have the following code segment :
What is the output of this code?
Reason — The + operator creates a new string by joining the two operand strings.
Question 12
Reason — string.upper() method returns a copy of the string converted to uppercase. The + operator creates a new string by joining the two operand strings.
Question 13
Which line of code produces an error ?
- "one" + 'two'
- "one" + "2"
Reason — The + operator has to have both operands of the same type either of number type (for addition) or of string type (for concatenation). It cannot work with one operand as string and one as a number.
Question 14
What is the output of this code ?
Reason — The + operator concatenates two strings and int converts it to integer type.
int("3" + "4") = int("34") = 34
Question 15
Which line of code will cause an error ?
Reason — Index 5 is out of range because list has 5 elements which counts to index 4.
Question 16
Which is the correct form of declaration of dictionary ?
Day = {1:'Monday', 2:'Tuesday', 3:'wednesday'}
- Day = {1;'Monday', 2;'Tuesday', 3;'wednesday'}
- Day = [1:'Monday', 2:'Tuesday', 3:'wednesday']
- Day = {1'monday', 2'tuesday', 3'wednesday'}
Reason — The syntax of dictionary declaration is:
According this syntax, Day = {1:'Monday', 2:'Tuesday', 3:'wednesday'} is the correct answer.
Question 17
Identify the valid declaration of L:
L = ['Mon', '23', 'hello', '60.5']
Reason — A list can store a sequence of values belonging to any data type and enclosed in square brackets.
Question 18
Suppose a tuple T is declared as T = (10, 12, 43, 39), which of the following is incorrect ?
- print(T[1])
- print(max(T))
- print(len(T))
Reason — Tuples are immutable. Hence we cannot perform item-assignment in tuples.
Fill in the Blanks
Strings in Python store their individual letters in Memory in contiguous location.
Operator + when used with two strings, gives a concatenated string.
Operator + when used with a string and an integer gives an error.
Part of a string containing some contiguous characters from the string is called string slice .
The * operator when used with a list/string and an integer, replicates the list/string.
Tuples or Strings are not mutable while lists are.
Using list() function, you can make a true copy of a list.
The pop() function is used to remove an item from a list/dictionary.
The del statement can remove an individual item or a slice from a list.
The clear() function removes all the elements of a list/dictionary.
Creating a tuple from a set of values is called packing .
Creating individual values from a tuple's elements is called unpacking .
The keys() method returns all the keys in a dictionary.
The values() function returns all values from Key : value pair of a dictionary.
The items() function returns all the Key : value pairs as (key, value) sequences.
True/False Questions
Do both the following represent the same list. ['a', 'b', 'c'] ['c', 'a', 'b']
Reason — Lists are ordered sequences. In the above two lists, even though the elements are same, they are at different indexes (i.e., different order). Hence, they are two different lists.
A list may contain any type of objects except another list.
Reason — A list can store any data types and even list can contain another list as element.
There is no conceptual limit to the size of a list.
Reason — The list can be of any size.
All elements in a list must be of the same type.
Reason — A list is a standard data type of python that can store a sequence of values belonging to any data type.
A given object may appear in a list more than once.
Reason — List can have duplicate values.
The keys of a dictionary must be of immutable types.
Reason — Dictionaries are indexed by keys. Hence its keys must be of any non-mutable type.
You can combine a numeric value and a string by using the + symbol.
Reason — The + operator has to have both operands of the same type either of number type (for addition) or both of string type (for concatenation). It cannot work with one operand as string and one as a number.
The clear( ) removes all the elements of a dictionary but does not delete the empty dictionary.
Reason — The clear() method removes all items from the dictionary and the dictionary becomes empty dictionary post this method. del statement removes the complete dictionary as an object.
The max( ) and min( ) when used with tuples, can work if elements of the tuple are all of the same type.
Reason — Tuples should contain same type of elements for max() and min() method to work.
A list of characters is similar to a string type.
Reason — In Python, a list of characters and a string type are not similar. Strings are immutable sequences of characters, while lists are mutable sequences that can contain various data types. Lists have specific methods and behaviors that differ from strings, such as append(), extend(), pop() etc.
For any index n, s[:n] + s[n:] will give you original string s.
Reason — s[:n] — The slicing of a string starts from index 0 and ends at index n-1. s[n:] — The slicing of a string starts from index n and continues until the end of the string. So when we concatenate these two substrings we get original string s.
A dictionary can contain keys of any valid Python types.
Reason — The keys of a dictionary must be of immutable types.
Assertions and Reasons
Assertion. Lists and Tuples are similar sequence types of Python, yet they are two different data types.
Reason. List sequences are mutable and Tuple sequences are immutable.
Both Assertion and Reason are true and Reason is the correct explanation of Assertion.
Explanation Lists and tuples are similar sequence types in python, but they are distinct data types. Both are used to store collections of items, but they have different properties and use cases. Lists are mutable, meaning you can add, remove, or modify elements after the list is created. Tuples, on the other hand, are immutable, meaning once a tuple is created, its contents cannot be changed.
Assertion. Modifying a string creates another string internally but modifying a list does not create a new list.
Reason. Strings store characters while lists can store any type of data.
Both Assertion and Reason are true but Reason is not the correct explanation of Assertion.
Explanation In Python, strings are immutable, meaning once they are created, their contents cannot be changed. Whenever we modify a string, python creates a new string object to hold the modified contents, leaving the original string unchanged. On the other hand, lists in python are mutable, meaning we can modify their contents after they have been created. When we modify a list, python does not create a new list object. Instead, it modifies the existing list object in place. Strings are sequences of characters, and each character in a string can be accessed by its index. Lists, on the other hand, can store any type of data, including integers, floats, strings.
Reason. Strings are immutable types while lists are mutable types of python.
Explanation In Python, strings are immutable, meaning once they are created, their contents cannot be changed. Whenever we modify a string, python creates a new string object to hold the modified contents, leaving the original string unchanged. On the other hand, lists in python are mutable, meaning we can modify their contents after they have been created. When we modify a list, python does not create a new list object. Instead, it modifies the existing list object in place.
Assertion. Dictionaries are mutable, hence its keys can be easily changed.
Reason. Mutability means a value can be changed in place without having to create new storage for the changed value.
Assertion is false but Reason is true.
Explanation Dictionaries are indexed by keys and each key must be immutable and unique. However, the dictionary itself is mutable, meaning that we can add, remove, or modify key-value pairs within the dictionary without changing the identity of the dictionary object itself. Mutability refers to the ability to change a value in place without creating a new storage location for the changed value.
Assertion. Dictionaries are mutable but their keys are immutable.
Reason. The values of a dictionary can change but keys of dictionary cannot be changed because through them data is hashed.
Explanation A dictionary is a unordered set of key : value pairs and are indexed by keys. The values of a dictionary can change but keys of dictionary cannot be changed because through them data is hashed. Hence dictionaries are mutable but keys are immutable and unique.
Assertion. In Insertion Sort, a part of the array is always sorted.
Reason. In Insertion sort, each successive element is picked and inserted at an appropriate position in the sorted part of the array.
Explanation Insertion sort is a sorting algorithm that builds a sorted list, one element at a time from the unsorted list by inserting the element at its correct position in sorted list. In Insertion sort, each successive element is picked and inserted at an appropriate position in the previously sorted array.
Type A: Short Answer Questions/Conceptual Questions
What is the internal structure of python strings ?
Strings in python are stored as individual characters in contiguous memory locations, with two-way index for each location. The index (also called subscript) is the numbered position of a letter in the string. Indices begin 0 onwards in the forward direction up to length-1 and -1,-2, .... up to -length in the backward direction. This is called two-way indexing.
Write a python script that traverses through an input string and prints its characters in different lines - two characters per line.
Discuss the utility and significance of Lists, briefly.
A list is a standard data type of python that can store a sequence of values belonging to any type. Lists are mutable i.e., we can change elements of a list in place. Their dynamic nature allows for flexible manipulation, including appending, inserting, removing, and slicing elements. Lists offer significant utility in data storage, iteration, and manipulation tasks.
What do you understand by mutability ? What does "in place" task mean ?
Mutability means that the value of an object can be updated by directly changing the contents of the memory location where the object is stored. There is no need to create another copy of the object in a new memory location with the updated values. Examples of mutable objects in python include lists, dictionaries. In python, "in place" tasks refer to operations that modify an object directly without creating a new object or allocating additional memory. For example, list methods like append(), extend(), and pop() perform operations in place, modifying the original list, while string methods like replace() do not modify the original string in place but instead create a new string with the desired changes.
Start with the list [8, 9, 10]. Do the following using list functions:
- Set the second entry (index 1) to 17
- Add 4, 5 and 6 to the end of the list
- Remove the first entry from the list
- Sort the list
- Double the list
- Insert 25 at index 3
listA = [8, 9, 10]
- listA[1] = 17
- listA.extend([4, 5, 6])
- listA.pop(0)
- listA.sort()
- listA = listA * 2
- listA.insert(3, 25)
What's a[1 : 1] if a is a string of at least two characters ? And what if string is shorter ?
a[x:y] returns a slice of the sequence from index x to y - 1. So, a[1 : 1] will return an empty list irrespective of whether the list has two elements or less as a slice from index 1 to index 0 is an invalid range.
What are the two ways to add something to a list ? How are they different ?
The two methods to add something to a list are:
append method — The syntax of append method is list.append(item) .
extend method — The syntax of extend method is list.extend(<list>) .
The difference between the append() and extend() methods in python is that append() adds one element at the end of a list, while extend() can add multiple elements, given in the form of a list, to a list.
append method:
Output — [10, 12, 14, 16]
extend method:
Output — ['a', 'b', 'c', 'd', 'e']
What are the two ways to remove something from a list? How are they different ?
The two ways to remove something from a list are:
pop method — The syntax of pop method is List.pop(<index>) .
del statement — The syntax of del statement is
The difference between the pop() and del is that pop() method is used to remove single item from the list, not list slices whereas del statement is used to remove an individual item, or to remove all items identified by a slice.
pop() method:
Output — 'k'
del statement:
Output — [1, 2, 5]
What is the difference between a list and a tuple ?
In the Python shell, do the following :
- Define a variable named states that is an empty list.
- Add 'Delhi' to the list.
- Now add 'Punjab' to the end of the list.
- Define a variable states2 that is initialized with 'Rajasthan', 'Gujarat', and 'Kerala'.
- Add 'Odisha' to the beginning of the list states2.
- Add 'Tripura' so that it is the third state in the list states2.
- Add 'Haryana' to the list states2 so that it appears before 'Gujarat'. Do this as if you DO NOT KNOW where 'Gujarat' is in the list. Hint. See what states2.index("Rajasthan") does. What can you conclude about what listname.index(item) does ?
- Remove the 5th state from the list states2 and print that state's name.
- states = []
- states.append('Delhi')
- states.append('Punjab')
- states2 = ['Rajasthan', 'Gujarat', 'Kerala']
- states2.insert(0,'Odisha')
- states2.insert(2,'Tripura')
- a = states2.index('Gujarat') states2.insert(a - 1,'Haryana')
- b = states2.pop(4) print(b)
Discuss the utility and significance of Tuples, briefly.
Tuples are used to store multiple items in a single variable. It is a collection which is ordered and immutable i.e., the elements of the tuple can't be changed in place. Tuples are useful when values to be stored are constant and need to be accessed quickly.
If a is (1, 2, 3)
- what is the difference (if any) between a * 3 and (a, a, a) ?
- Is a * 3 equivalent to a + a + a ?
- what is the meaning of a[1:1] ?
- what is the difference between a[1:2] and a[1:1] ?
- a * 3 ⇒ (1, 2, 3, 1, 2, 3, 1, 2, 3) (a, a, a) ⇒ ((1, 2, 3), (1, 2, 3), (1, 2, 3)) So, a * 3 repeats the elements of the tuple whereas (a, a, a) creates nested tuple.
- Yes, both a * 3 and a + a + a will result in (1, 2, 3, 1, 2, 3, 1, 2, 3).
- This colon indicates (:) simple slicing operator. Tuple slicing is basically used to obtain a range of items. tuple[Start : Stop] ⇒ returns the portion of the tuple from index Start to index Stop (excluding element at stop). a[1:1] ⇒ This will return empty list as a slice from index 1 to index 0 is an invalid range.
- Both are creating tuple slice with elements falling between indexes start and stop. a[1:2] ⇒ (2,) It will return elements from index 1 to index 2 (excluding element at 2). a[1:1] ⇒ () a[1:1] specifies an invalid range as start and stop indexes are the same. Hence, it will return an empty list.
What is the difference between (30) and (30,) ?
a = (30) ⇒ It will be treated as an integer expression, hence a stores an integer 30, not a tuple. a = (30,) ⇒ It is considered as single element tuple since a comma is added after the element to convert it into a tuple.
Write a Python statement to declare a Dictionary named ClassRoll with Keys as 1, 2, 3 and corresponding values as 'Reena', 'Rakesh', 'Zareen' respectively.
Why is a dictionary termed as an unordered collection of objects ?
A dictionary is termed as an unordered collection of objects because the elements in a dictionary are not stored in any particular order. Unlike string, list and tuple, a dictionary is not a sequence. For a dictionary, the printed order of elements is not the same as the order in which the elements are stored.
What type of objects can be used as keys in dictionaries ?
Keys of a dictionary must be of immutable types such as
- a Python string
- a tuple (containing only immutable entries)
Though tuples are immutable type, yet they cannot always be used as keys in a dictionary. What is the condition to use tuples as a key in a dictionary ?
For a tuple to be used as a key in a dictionary, all its elements must be immutable as well. If a tuple contains mutable elements, such as lists, sets, or other dictionaries, it cannot be used as a key in a dictionary.
Dictionary is a mutable type, which means you can modify its contents ? What all is modifiable in a dictionary ? Can you modify the keys of a dictionary ?
Yes, we can modify the contents of a dictionary. Values of key-value pairs are modifiable in dictionary. New key-value pairs can also be added to an existing dictionary and existing key-value pairs can be removed. However, the keys of the dictionary cannot be changed. Instead we can add a new key : value pair with the desired key and delete the previous one. For example:
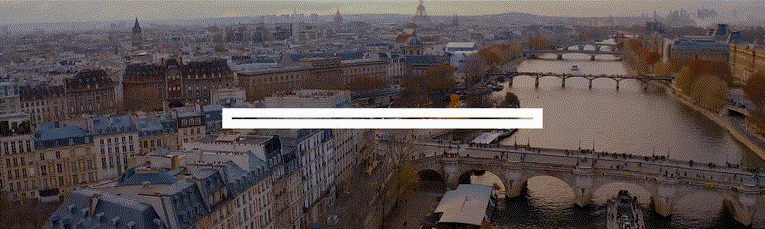
Explanation
d is a dictionary which contains one key-value pair. d[2] = 2 adds new key-value pair to d. d[1] = 3 modifies value of key 1 from 1 to 3. d[3] = 2 adds new key-value pair to d. del d[2] deletes the key 2 and its corresponding value.
Question 19
How is del D and del D[<key>] different from one another if D is a dictionary ?
del D deletes the entire dictionary D. After executing del D , the variable D is no longer defined, and any attempt to access D will result in a NameError . del D[<key>] deletes the key-value pair associated with the specified key from the dictionary D . After executing del D[<key>] , the dictionary D still exists, but the specified key and its corresponding value are removed from the dictionary.
For example:
Question 20
Create a dictionary named D with three entries, for keys 'a', 'b' and 'c'. What happens if you try to index a nonexistent key (D['d']) ? What does python do if you try to assign to a nonexistent key d. (e.g., D['d'] = 'spam') ?
- In this example, the dictionary D does not contain the key 'd'. Therefore, attempting to access this key by D['d'] results in a KeyError because the key does not exist in the dictionary.
- If we try to assign a value to a nonexistent key in a dictionary, python will create that key-value pair in the dictionary. In this example, the key 'd' did not previously exist in the dictionary D. When we attempted to assign the value 'spam' to the key 'd', python created a new key-value pair 'd': 'spam' in the dictionary D.
Question 21
What is sorting ? Name some popular sorting techniques.
Sorting refers to arranging elements of a sequence in a specific order — ascending or descending.
Sorting Techniques are as follows :
- Bubble Sort
- Insertion Sort
- Selection Sort
Question 22
Discuss Bubble sort and Insertion sort techniques.
Bubble Sort : In Bubble sort, the adjoining values are compared and exchanged if they are not in proper order. This process is repeated until the entire array is sorted.
Insertion Sort : Insertion sort is a sorting algorithm that builds a sorted list one element at a time from the unsorted list by inserting the element at its correct position in sorted list.
Type B: Application Based Questions
Question 1(a).
What will be the output produced by following code fragments ?
str(123) converts the number 123 to string and stores in y so y becomes "123". "hello" * 3 repeats "hello" 3 times and stores it in x so x becomes "hellohellohello".
"hello" + "world" concatenates both the strings so x becomes "helloworld". As "helloworld" contains 10 characters so len(x) returns 10.
Question 1(b)
The code concatenates three strings "hello", "to Python", and "world" into the variable x . Then, it iterates over each character in x using a for loop. For each character, it assigns it to the variable y and prints y followed by a colon and space, all on the same line due to the end=" " parameter.
Question 1(c)
print(x[:2], x[:-2], x[-2:]) — x[:2] extracts the substring from the beginning of x up to index 1, resulting in "he". x[:-2] extracts the substring from the beginning of x up to the third last character, resulting in "hello wor". x[-2:] extracts the substring from the last two characters of x until the end, resulting in "ld". Hence, output of this line becomes he hello wor ld
print(x[6], x[2:4]) — x[6] retrieves the character at index 6, which is 'w'. x[2:4] extracts the substring from index 2 up to index 3, resulting in "ll". Hence, output of this line becomes w ll
print(x[2:-3], x[-4:-2]) — x[2:-3] extracts the substring from index 2 up to the fourth last character, resulting in "llo wo". x[-4:-2] extracts the substring from the fourth last character to the third last character, resulting in "or". Hence, output of this line becomes llo wo or
Write a short Python code segment that adds up the lengths of all the words in a list and then prints the average (mean) length.
- The code prompts the user to enter a list of words and assigns it to the variable word_list .
- We iterate over word_list using for loop. Inside the loop, length of each word gets added to total_length variable.
- Average length is calculated by dividing total_length by the number of words in word_list .
Predict the output of the following code snippet ?
The slicing notation a[start:stop:step] extracts a portion of the list from index start to stop-1 with a specified step. In the slicing part a[3:0:-1] :
- start is 3, which corresponds to the element with value 4.
- stop is 0, but as element at stop index is excluded so slicing goes up to index 1.
- step is -1, indicating that we want to step backward through the list.
Putting it together:
This extracts elements from index 3 to (0+1) in reverse order with a step of -1.
The output of the code will be:
Question 4(a)
Predict the output of the following code snippet?
- arr is initialised as a list with elements [1, 2, 3, 4, 5, 6].
- for loop iterates over the indices from 1 to 5. For each index i , it assigns the value of arr[i] to arr[i - 1] , effectively shifting each element one position to the left. After this loop, the list arr becomes [2, 3, 4, 5, 6, 6] .
- Second for loop iterates over the indices from 0 to 5 and prints each element of the list arr without newline characters because of end="" parameter. Then it prints the elements of the modified list arr , resulting in 234566 .
Question 4(b)
- Numbers is a list containing the numbers 9, 18, 27, and 36.
- The outer for loop iterates over each element in the list Numbers .
- The inner loop iterates over the range from 1 to the remainder of Num divided by 8. For example, if Num is 9, the range will be from 1 to 1 (9 % 8 = 1). If Num is 18, the range will be from 1 to 2 (18 % 8 = 2), and so on. Then it prints the value of N, followed by a "#", and ensures that the output is printed on the same line by setting end=" ".
- After both loops, it prints an empty line, effectively adding a newline character to the output.
Question 5(a)
Find the errors. State reasons.
t[0] = 6 will raise a TypeError as tuples are immutable (i.e., their elements cannot be changed after creation).
Question 5(b)
There are no errors in this python code. Lists in python can contain elements of any type. As lists are mutable so t[0] = 6 is also valid.
Question 5(c)
t[4] = 6 will raise an error as we are trying to change the value at index 4 but it is outside the current range of the list t . As t has 3 elements so its indexes are 0, 1, 2 only.
Question 5(d)
t[0] = "H" will raise an error because strings in python are immutable, meaning we cannot change individual characters in a string after it has been created. Therefore, attempting to assign a new value to t[0] will result in an error.
Question 5(e)
The errors in this code are:
- In the list [Amar, Shveta, Parag] , each element should be enclosed in quotes because they are strings.
- The equality comparison operator is '==' instead of = for checking equality.
- if statement should be lowercase.
Assuming words is a valid list of words, the program below tries to print the list in reverse. Does it have an error ? If so, why ? (Hint. There are two problems with the code.)
There are two issue in range(len(words), 0, -1) :
- The start index len(words) is invalid for the list words as it will have indexes from 0 to len(words) - 1 .
- The end index being 0 means that the last element of the list is missed as the list will be iterated till index 1 only.
The corrected python code is :
What would be the output of following code if ntpl = ("Hello", "Nita", "How's", "life ?") ?
ntpl is a tuple containing 4 elements. The statement (a, b, c, d) = ntpl unpacks the tuple ntpl into the variables a, b, c, d. After that, the values of the variables are printed.
The statement ntpl = (a, b, c, d) forms a tuple with values of variables a, b, c, d and assigns it to ntpl. As these variables were not modified, so effectively ntpl still contains the same values as in the first statement.
ntpl[0] ⇒ "Hello" ∴ ntpl[0][0] ⇒ "H"
ntpl[1] ⇒ "Nita" ∴ ntpl[1][1] ⇒"i"
ntpl[0][0] and ntpl[1][1] concatenates to form "Hi". Thus ntpl[0][0]+ntpl[1][1], ntpl[1] will return "Hi Nita ".
What will be the output of the following code ?
Tuples can be declared with or without parentheses (parentheses are optional). Here, tuple_a is declared without parentheses where as tuple_b is declared with parentheses but both are identical. As both the tuples contain same values so the equality operator ( == ) returns true.
What will be the output of the following code snippet ?
In the given python code snippet, id1 and id2 will point to two different objects in memory as del rec deleted the original dictionary whose id is stored in id1 and created a new dictionary with the same contents storing its id in id2 . However, id1 == id2 will compare the contents of the two dictionaries pointed to by id1 and id2 . As contents of both the dictionaries are same hence it returns True . If in this code we add another line print(id1 is id2) then this line will print False as id1 and id2 point to two different dictionary objects in memory.
Write the output of the code given below :
A dictionary my_dict with two key-value pairs, 'name': 'Aman' and 'age': 26 is initialized. Then updates the value associated with the key 'age' to 27. Then adds a new key-value pair 'address': 'Delhi' to the dictionary my_dict . The items() method returns all of the items in the dictionary as a sequence of (key, value) tuples. In this case, it will print [('name', 'Aman'), ('age', 27), ('address', 'Delhi')].
Write a method in python to display the elements of list thrice if it is a number and display the element terminated with '#' if it is not number.
For example, if the content of list is as follows :
The output should be 414141 DROND# GIRIRAJ# 131313 ZAR#
- The code prompts the user to enter the elements of the list separated by spaces and stores the input as a single string in the variable my_list .
- Then splits the input string my_list into individual elements and stores them in a new list called new_list .
- Then for loop iterates over each element in the new_list.
- The isdigit() method is used to check if all characters in the string are digits. If it's true (i.e., if the element consists only of digits), then it prints the element concatenated with itself three times. Otherwise, if the element contains non-digit characters, it prints the element concatenated with the character '#'.
Name the function/method required to
(i) check if a string contains only uppercase letters.
(ii) gives the total length of the list.
(i) isupper() method is used to check if a string contains only uppercase letters.
(ii) len() gives the total length of the list.
- An empty dictionary named my_dict is initialized.
- my_dict[(1,2,4)] = 8, my_dict[(4,2,1)] = 10, my_dict[(1,2)] = 12 these lines assign values to the dictionary my_dict with keys as tuples. Since tuples are immutable, so they can be used as keys in the dictionary.
- The for loop iterates over the keys of the dictionary my_dict . Inside the loop, the value associated with each key k is added to the variable sum .
- sum and my_dict are printed.
Type C: Programming Practice/Knowledge based Questions
Write a program that prompts for a phone number of 10 digits and two dashes, with dashes after the area code and the next three numbers. For example, 017-555-1212 is a legal input. Display if the phone number entered is valid format or not and display if the phone number is valid or not (i.e., contains just the digits and dash at specific places).
Write a program that should prompt the user to type some sentence(s) followed by "enter". It should then print the original sentence(s) and the following statistics relating to the sentence(s):
- Number of words
- Number of characters (including white-space and punctuation)
- Percentage of characters that are alpha numeric
- Assume any consecutive sequence of non-blank characters in a word.
Write a program that takes any two lists L and M of the same size and adds their elements together to form a new list N whose elements are sums of the corresponding elements in L and M. For instance, if L = [3, 1, 4] and M = [1, 5, 9], then N should equal [4, 6, 13].
Write a program that rotates the elements of a list so that the element at the first index moves to the second index, the element in the second index moves to the third index, etc., and the element in the last index moves to the first index.
Write a short python code segment that prints the longest word in a list of words.
Write a program that creates a list of all the integers less than 100 that are multiples of 3 or 5.
Define two variables first and second so that first = "Jimmy" and second = "Johny". Write a short python code segment that swaps the values assigned to these two variables and prints the results.
Write a python program that creates a tuple storing first 9 terms of Fibonacci series.
Create a dictionary whose keys are month names and whose values are the number of days in the corresponding months.
(a) Ask the user to enter a month name and use the dictionary to tell them how many days are in the month.
(b) Print out all of the keys in alphabetical order.
(c) Print out all of the months with 31 days.
(d) Print out the (key-value) pairs sorted by the number of days in each month.
Write a function called addDict(dict1, dict2) which computes the union of two dictionaries. It should return a new dictionary, with all the items in both its arguments (assumed to be dictionaries). If the same key appears in both arguments, feel free to pick a value from either.
Write a program to sort a dictionary's keys using Bubble sort and produce the sorted keys as a list.
Write a program to sort a dictionary's values using Bubble sort and produce the sorted values as a list.
Coding Python
Python Revision Tour Worksheet Class 12
Multiple Choice Questions: (20 x 1=20)
1. What will be the output of the following Python code?
from math import factorial print (math.factorial (5))
(a) 120 (b) Nothing is printed (c) Error, method factorial doesn’t exist in math module (d) Error, the statement should be: print(factorial(5))
2. What is the order of namespaces in which Python looks for an identifier? (a) Python first searches the global namespace, then the local namespace and finally the built-in namespace (b) Python first searches the local namespace, then the global namespace and finally the built-in namespace (c) Python first searches the built-in namespace, then the global namespace and finally the local namespace (d) Python first searches the built-in namespace, then the local namespace and finally the gloab namespace
3. Which of the following is not a valid namespace? (a) Global namespace (b) Public namespace (c) Built-in namespace (d) Local namespace
4. What will be the output of the following Python code?
#modules def change (a): b=[x * 2 for x in a] print (b)
#module 2 def change (a): b= (x*x for x in a) print (b)
from module import change from module2 import change #main s = [1, 2, 3] change (s)
(a) [2, 4, 6] (b) [1, 4, 9] (c) [2, 4, 6] [1, 4, 9] (d) There is a name cash
5. What is the output of the function shown below (random module has already been imported)?
random.choice(‘sun’)
(a) sun (b) u (c) either s, u or n (d) error
6. What possible output(s) are expected to be displayed on screen at the time of execution of the program from the following code?
import random AR = [ 20, 30, 40, 50, 60, 70] FROM = random.randint (1, 3) TO = random.randint (2, 4) for K in range (FROM, TO+1): print (AR(K), end = “#”)
(a) 10#40#70# (b) 30#40#50# (c) 50#60#70# (d) 40#50#70#
7. Which of the statements is used to import all names from a module into the current calling module? (a) import (b) from (c) import * (d) dir()
8. Which of the variables tells the interpreter where to locate the module files imported into a program? (a) local (b) import variable (c) PYTHONPATH (d) current
9. Which of the following date class function returns the current system date? (a) day() (b) today() (c) month() (d) year()
10. What is the range of values that random.random() can return? (a) [0.0, 1.0] (b) (0.0, 1.0] (c) (0.0, 1.0) (d) [0.0, 1.0)
11. A .py file containing constants/variables, classes, functions etc. related to a particular task and can be used in other programs is called (a) module (b) library (c) classes (d) documentation
12. The collection of modules and packages that together cater to a specific type of applications or requirements, is called ____. (a) module (b) library (c) classes (d) documentation
13. An independent triple quoted string given inside a module, containing documentation related information is a ____. (a) Documentation string (b) docstring (c) dstring (d) stringdoc
14. The help <module> statement displays ____ from a module. (a) constants (b) functions (c) classes (d) docstrings
15. Which command(s) modifies the current namespace with the imported object name? (a) import <module> (b) import <module1>, <module2> (c) from <module> import <object> (d) from <module> import *
16. Which command(s) creates a separate namespace for each of the imported module? (a) import <module> (b) import <module1>, <module2> (c) from <module> import <object> (d) from <module> import *
17. Which of the following random module functions generates a floating point number? (a) random() (b) randint() (c) uniform() (d) all of these
18. Which of the following random module functions generates an integer? (a) random() (b) randint() (c) uniform() (d) all of these
19. Which file must be a part of a folder to be used as a Python package? (a) package.py (b) __init__.py (c) __package__.py (d) __module.py__
20. A Python module has ___ extension. (a) .mod (b) .imp (c).py (d) .mpy
Fill in the Blanks:(5 x 1=5)
21. Commonly-used modules that contain source code for generic needs are called _____.
22. A Python _____ is a directory of Python module(s).
23 We can use any Python source file as a module by executing an _____ statement
24._______ are used to distinguish between different sections of a program.
25. A _____ is a separately saved unit whose functionality can be reused at will.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.

Python Revision Tour Class 12 Notes | CBSE Computer Science
Python Revision Tour Class 12 Notes covers Python Fundamentals of class 11 including Variables, Operators, Input and Output, Flow of control, Expressions, Type Casting, Strings, List, Tuples, Dictionary. All the concepts are explained with examples so that students can build strong foundation in Python Programming Fundamentals.
- 1 Getting Started with Python
- 5 Dictionary
Getting Started with Python
Python is a General Purpose high level Programming language used for developing application softwares.
Features of Python
- Free and Open Source
- Case Sensitive
- Interpreted
- Plateform Independent
- Rich library of functions
- General Purpose
Working with Python
To write and run python programs we need Python Interpreter also called Python IDLE.
Execution Mode
We can use Python Interpreter in two ways:
- Interactive mode
- Script mode
Interactive Mode
- Instant execution of individual statement
- Convenient for testing single line of code
- We cannot save statements for future use
Script Mode
- Allows us to write and execute more than one Instruction together.
- We can save programs (python script) for future use
- Python scripts are saved as file with extension “.py”
How to execute or run python program
- Open python IDLE
- Click ‘File’ and select ‘New’ to open Script mode
- Type source code and save it
- Click on ‘Run’ menu and select ‘Run Module’

Python Keywords
- These are predefined words which a specific meaning to Python Interpreter.
- These are reserve keywords
- Keywords in python are case sensitive

Identifiers are name used to identify a variable, function or any other entities in a programs.
Rules for naming Identifier
- The name should begin with an alphabet or and underscore sign and can be followed by any combination of charaters a-z, A-Z, 0-9 or underscore.
- It can be of any length but we should keep it simple, short and meaningful.
- it should not be a python keyword or reserved word.
- We cannot use special symbols like !, @, #, $, % etc. in identifiers.
- It can be referred as an object or element that occupies memory space which can contain a value.
- Value of variable can be numeric, alphanumeric or combination of both.
- In python assignment statement is used to create variable and assign values to it.
These are keywords which determine the type of data stored in a variable. Following table show data types used in python:

- These are statement ignored by python interpreter during execution.
- It is used add a remark or note in the source code.
- It starts with # (hash sign) in python.
These are special symbols used to perform specific operation on values. Different types of operator supported in python are given below:
- Arithmetic operator
- Relational operator
- Assignment operator
- Logical operator
- Identity operator
- Membership operator

Expressions
- An expression is combination of different variables, operators and constant which is always evaluated to a value.
- A value or a standalone variable is also considered as an expression.
56 + (23-13) + 89%8 – 2*3
Evaluation:
= 56 + (23 -13) + 89%8 – 2*3 #step1 = 56 + 10 + (89%8) – 2*3 #step2 = 56 + 10 + 1 – (2*3) #step3 = (56 + 10) + 1 – 6 #step4 = (66 + 1) – 6 #step5 = 67 – 6 #step6 = 61
A statement is unit of code that the python interpreter can execute.
var1 = var2 #assignment statement x = input (“enter a number”) #input statement print (“total = “, R) #output statement
How to input values in python?
In python we have input() function for taking user input.
Input([prompt])
How to display output in python ?
In python we have print() function to display output.
print([message/value])
Python program to input and output your name
var = input(“Enter your name”) print(“Name you have entered is “, var)
Addition of two numbers
Var1 = int(input(“enter no1”)) Var2 = int(input(“enter no2”)) Total = Var1 + Var2 Print(“Total = “, Total)
Type Conversion
Type conversion refers to converting one type of data to another type.
Type conversion can happen in two ways:
- Explicit conversion
Implicit conversion
Explicit Conversion
- Explicit conversion also refers to type casting.
- In explicit conversion, data type conversion is forced by programmer in program
(new_data_type) = (expression) Explicit type conversion function

Program of explicit type conversion from float to int
x = 12 y = 5 print(x/y) #output – 2.4 print(int(x/y)) #output – 2
Program of explicit type conversion from string to int
x = input(“enter a number”) print(x+2) #output – produce error “can only concatenate str to str x = int (input(Enter a number”)) print(x+2) #output – will display addition of value of x and 2
- Implicit conversion is also known as coercion.
- In implicit conversion data type conversion is done automatically.
- Implicit conversion allows conversion from smaller data type to wider size data type without any loss of information
Program to show implicit conversion from int to float
var1 = 10 #var1 is integer var2 = 3.4 #var2 is float res = var1 – var2 #res becomes float automatically after subtraction print(res) #output – 6.6 print(type(res)) #output – class ‘Float’
- String is basically a sequence which is made up of one or more UNICODE characters.
- Character in string can be any letter, digit, whitespace or any other symbol.
- String can be created by enclosing one or more characters in single, double or triple quotes.
Accessing characters in a string (INDEX)
- Individual character in a string can be accessed using indexes.
- Indexes are unique numbers assigned to each character in a string to identify them
- Index always begins from 0 and written in square brackets “[]”.
- Index must be an zero, positive or negative integer.
- We get IndexError when we give index value out of the range.
Negative Index
- Python allows negative indexing also.
- Negative indices are used when you want to access string in reverse order.
- Starting from the right side, the first character has the index as -1 and the last character (leftmost) has the index –n where n is length of string.
Is string immutable?
- Yes, string is immutable data type. The content of string once assigned cannot be altered than.
- Trying to alter string content may lead an error.
String operations
String supports following operations:
Concatenation
- Concatenation refers to joining two strings.
- Plus (‘+’) is used as concatenation operator.
- Repetition as it name implies repeat the given string.
- Asterisk (‘*’) is used as repetition operator.
- Membership operation refers to checking a string or character is part or subpart of an existing string or not.
- Python uses ‘in’ and ‘not in’ as membership operator.
- ‘in’ returns true if the first string or character appears as substring in the second string.
- ‘not in’ returns true if the first string or character does not appears as substring in the second string.
- Extracting a specific part of string or substring is called slicing
- Subset occurred after slicing contains contiguous elements
- Slicing is done using index range like string[start_index : end_index : step_value]
- End index is always excluded in resultant substring.
- Negative index can also be used for slicing.
Traversing a String
- Traversing a string refers to accessing each character of a given string sequentially.
- For or while loop is used for traversing a string
String Functions
- List is built in sequence data type in python.
- Stores multiple values
- List item can be of different data types
- All the items are comma separated and enclosed in square bracket.
- Individual item in a list can be accessed using index which begins from 0.

Accessing elements in a List
- Individual item in a list can be accessed using indexes.
- Indexes are unique numbers assigned to each item in a list to identify them

List is Mutable
- Yes list is mutable.
- Content of the list can be changed after it has been created.

List operations
List supports following operations:
- Concatenation refers to joining two List.
- Concatenating List with other data type produces TypeErrors.

- Repetition as it name implies used to replicate a list at specified no of times.

- Membership operation refers to checking an item is exists in the list or not.
- ‘in’ returns true if the item specified present in the list.
- ‘not in’ returns true if the item specified present in the list.

- Extracting a subset of items from given list is called slicing
- Subset occurred after slicing contains contiguous items.
- Slicing is done using index range like List[start_index : end_index : step_value]
- End index is always excluded in resultant subset of list.

Traversing a List
- Traversing a List refers to accessing each item a given list sequentially.
- for or while loop can be used for traversing a list.

List methods | List Functions

Nested List
One list appears as an element inside another list.

- Tuple is built in sequence data type in python.
- Tuple item can be of different data types
- All the items are comma separated and enclosed in parenthesis ‘()’.
- Individual item in a Tuple can be accessed using index which begins from 0.
- In case of single item present in tuple, it should also be followed by a comma.
- A sequence without parenthesis is treated as tuple by default.

Accessing elements in a Tuple
- Individual item in a Tuple can be accessed using indexes.
- Indexes are unique numbers assigned to each item in a Tuple to identify them

Tuple is Immutable
- Yes Tuple is Immutable.
- Content of the Tuple cannot be changed after it has been created.

Tuple operations
Tuple supports following operations:
- Concatenation refers to joining two Tuple.
- Concatenation operator can also be used for extending an existing tuple.

- Repetition as it name implies used to repeat elements of a Tuple at specified no of times.

- Membership operation refers to checking an item exists in Tuple or not.
- ‘in’ returns true if the item specified present in the Tuple.
- ‘not in’ returns true if the item specified present in the Tuple.

- Extracting a subset of items from given Tuple is called slicing
- Slicing is done using index range like Tuple[start_index : end_index : step_value]
- End index is always excluded in resultant subset of Tuple.

Traversing a Tuple
- Traversing a Tuple refers to accessing each item a given Tuple sequentially.
- for or while loop can be used for traversing a Tuple.

Tuple Methods and Built in Functions

Nested Tuple
One Tuple appears as an element inside another Tuple.

Tuple Assignment
Tuple Assignment allows elements of tuple on the left side of assignment operator to be assigned respective values from a tuple on the right side.

- Dictionaries are unordered collection of items falls under mapping.
- Stores data in form of key:value pair called item.
- Key is separated from its value by colon ‘:’ and items are separated by comma.
- All items of dictionaries are enclosed in curly bracket ‘{}’.
- The keys in the dictionary must be unique and should be of immutable data type.
- The value can be repeated and of any data type.
Creating a Dictionary

Accessing items in a Dictionary
- Items of dictionary are accessed using keys.
- Each key of dictionary serve as index and maps to a value.
- If key is not present in dictionary, then we get KeyError.

Dictionary is Mutable
Yes, Dictionary is Mutable as its content can be changed after it has been created.
Adding a new item in Dictionary

Modifying an existing Item in Dictionary

Traversing a dictionary

Dictionary methods and built in functions

1 thought on “Python Revision Tour Class 12 Notes | CBSE Computer Science”
Sir, how can I get the pdf form of your notes
Leave a Comment Cancel Reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Python revision Tour || Multiple Choice Questions || Class 12
1. Which of the following is an invalid variable?
(a) my_day_2
(b) 2nd_day
2. Which of the following is not a keyword?
(c) nonlocal
3. Which of the following cannot be a variable?
(a) __init__
4. Which of these is not a core data type?
(b) Dictionary
5. How would you write xy in Python as an expression?
(d) none of these
6. What will be the value of the expression?
14 +13 % 15
7. Evaluate the expression given below if A = 16 and B = 15.
8. What is the value of x?
x = int(13.25 4/2)
9. The expression 8/4/2 will evaluate equivalent to which of the following expressions:
(a) 8/(4/2)
(b) (8/4)/2
10. Which among the following list of operators has the highest precedence?
+ ,-,**,%, /,<< ,>> , |
(a) <<, >>
11. Which of the following expressions results in an error?
(a) float('12')
(b) int('12')
(c) float('12.5')
(d) int('12.5')
12. Which of the following statement prints the shown output below?
hello\example\test.txt
(a) print("hello\example\test.txt")
(b) print("hello\\example\\test.txt")
(c) print("hello\"example \"test.txt")
(d) print("hello"\example"\test.txt")
13. Which value type does input() return?
(a) Boolean
14. Which two operators can be used on numeric values in Python?
15. Which of the following four code fragments will yield following output?
Select all of the function calls that result in this output
print('''Eina
(b) print('''EinaMinaDika''')
(c) print('Eina\nMina\nDika')
print('Eina
16. Which of the following is valid arithmetic operator in Python:
17. The numbered position of a letter in a string is called _____.
(a) position
(b) integer position
(d) location
18. The operator _____ tells if an element is present in a sequence or not.
19. The keys of a dictionary must be of _____ types.
(a) Integer
(b) mutable
(c) immutable
(d) any of these
20. Following set of commands is executed in shell, what will be the output?
>>>str = "hello"
>>>str[ : 2]
>>>
21. What data type is the object below?
L = [1, 23, 'hello', 1]
(b) dictionary
22. What data type is the object below?
L = 1, 23, 'hello', 1
23. To store values in terms of key and value, what core data type does Python provide?
(d) dictionary
24. What is the value of the following expression?
3 + 3.00, 3**3.0
(a) (6.0, 27.0)
(b) (6.0, 9.00)
(c) (6, 27)
(d) [6.0, 27.0]
(e) [6, 27]
25. List AL is defined as follows:
AL = [1, 2, 3, 4, 5]
Which of the following statements removes the middle element 3 from it so that the list AL equal [1, 2, 4, 5]?
(a) del a[2]
(b) a[2 : 3] = []
(c) a[2:2] = []
(d) a[2] = []
(e) a.remove(3)
26. Which two lines of code are valid strings in Python?
(a) This is a string
(b) 'This is a string'
(c) (This is a string)
(d) "This is a string"
27. You have the following code segment:
String1 = "my"
String2 = "work"
print(String1 + string2)
What is the output of this code?
(a) my work
28. You have the following code segment
print(String1 + string2.upper())
(b) MY Work
(d) My Work
29. Which line of code produces an error?
(a) "one" + 'two'
(c) "one"+ "2"
(d) '1' + 2
30. What is the output of this code?
>>> int("3" + " 4")
31. Which line of code will cause an error?
1. num = [5, 4, 3, [2], 1]
2. print(num [0])
3. print(num[3][0])
4. print (num[5])
32. Which is the correct form of declaration of dictionary?
(a) Day = {1 : 'Monday', 2 : 'Tuesday', 3 : 'wednesday'}
(b) Day = {1 ; 'Monday', 2 ; 'Tuesday', 3 ; 'wednesday'}
(c) Day = [1 : 'Monday', 2 : 'Tuesday', 3 : 'wednesday']
(d) Day = {1 'Monday', 2 'Tuesday', 3 'wednesday'}
33. Identify the valid declaration of L:
L = [1, 23, 'hi', 6]
34. Which of the following is not considered a valid identifier in Python?
(c) hello_rsp1
(d) 2 hundred
35. What will be the output of the following code-print("100+200")?
(c) 100+200
36. Which amongst the following is a mutable data type in Python?
37. pow() function belongs to which library?
38. Which of the following statements converts a tuple into a list?
(a) len(string)
(b) list(string)
(c) tup(list)
(d) dict(string)
39. The statement: bval = str i > str2 shall return..... As the output if two strings str1 and str2 contains "Delhi" and "New Delhi".
(c) New Delhi
40. What will be the output generated by the following snippet?
a = [5, 10, 15, 20, 25]
i = a[1] + 1
j = a[2] +1
print (i, j, m)
(a) 11 15 16
(b) 11 16 15
(c) 11 15 15
(d) 16 11 15
41. The process of arranging the array elements in a specified order is termed as:
(a) Indexing
(b) Slicing
(c) Sorting
(d) Traversing
42. Which of the following Python functions is used to iterate over a sequence of number by specifying a numeric end value within its parameters?
(a) range()
(c) substring()
(d) random()
43. What is the output of the following?
d = (0: 'a', 1: 'b', 2: 'c'}
for i in d:
44. What is the output of the following?
for i in x:
(d) infinite loop
45. Which arithmetic operators cannot be used with strings?
(d) all of the above
46. What will be the output when the following code is executed?
>>> str1="helloworld"
>>> stri[:-1]
(a) dlrowolleh
(d) helloworld
47. What is the output of the following statement?
print ("xyyzxyzxzxyy".count ('yy', 1))
48. Suppose list1 [0.5 * x for x in range(0, 4)], list1 is:
(a) [0, 1, 2, 3]
(b) [0, 1, 2, 3, 4]
(c) [0.0, 0.5, 1.0, 1.5]
(d) [0.0, 0.5, 1.0, 1.5, 20]
49. Which is the correct form of declaration of dictionary?
(a) Day={1:'monday',2:'tuesday',3:'wednesday'}
(b) Day=(1;'monday',2;'tuesday',3;'wednesday')
(c) Day=[1:'monday',2:'tuesday',3:'wednesday']
(d) Day={1 monday',2 tuesday',3 wednesday']
50. Identify the valid declaration of L: L = [1, 23, 'hi', 6]
You can help us by Clicking on ads. ^_^ Please do not send spam comment : )

thnak you, is it 2021-22?
bro i specially came to comment here..why are most of the answers given here in this MCQ part are wrong...fill in the blanks and true or false, both were fine..plz look into it
EDIT: I just noticed a pattern, the answers in itself aren't wrong..its just that, the numbering is wrong. For eg- In Q36. the answer is b) list, but the solution provided is d), this d) part is the answer of Q35..similarly in Q37. the answer is a) part, but the solution provided is D), this d) is the answer of previous question..in simple words answer of Q9 was not present but it got shifted to Q10, and answer of Q10 got shifted to Q11...and so on

We are Sorry for your inconvenience . Now we have fixed all problem.
This is a very good program. This has helped me a lot.
Post a Comment
Contact form.

+918076665624
Learnpython4cbse, inspiring success, click here for, vide o tutorial @ youtube channel supernova, there’s nothing here....
We can’t find the page you’re looking for. Check the URL, or head back home.
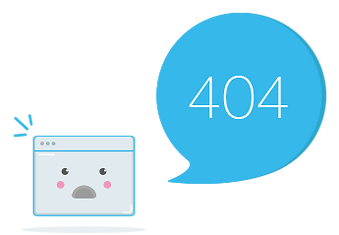
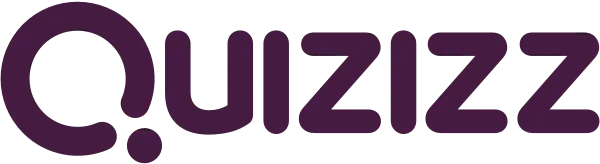
Have an account?

Python Revision Tour - II
15 questions
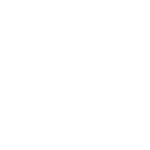
Introducing new Paper mode
No student devices needed. Know more
If you want to write code to check whether the given no. is divisible by 3. what condition will you write with if ( ) in the code written below
Identify the suitable construct to make the following Program
Identify the type of structure in the following code snippet
nested if else
(5==5) or (3==1) will give result
(4==5) or (3==3) will give result
(6) and (37==8) will give result
(8==8) and 6 will give result
(8!=8) and 6 will give result
(8) and 6==6 will give result
'Classroom Diary' or "Python" will produce result
Classroom Diary
( "Learn Python" and " " ) will produce result
Learn Python
( "Learn Python" and 2<3 ) will produce result
not ( "Learn Python" and 2<3 ) will produce result
In the expression x= 5/2 .....Data type of x is
Data type of "TRUE" is
Explore all questions with a free account
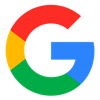
Continue with email
Continue with phone

MCQ for Class 12 Computer Science Python
Teachers and Examiners ( CBSESkillEduction ) collaborated to create the MCQ for Class 12 Computer Science Python. All the important Information are taken from the NCERT Textbook Computer Science (083) class 12 .
Computer Science Class 12 Notes
- Python Revision tour – 1 Class 12 Notes
- Python Revision tour – 2 Class 12 Notes Notes
- Working with Functions Class 12 Notes
- Using Python Libraries Class 12 Notes
- File Handling Class 12 Notes
- Recursion Class 12 Notes
- Idea of Algorithmic Efficiency Class 12 Notes
- Data Visualization using Pyplot Class 12 Notes
- Data Structure Class 12 Notes
- Computer Network Class 12 Notes
- More on MySQL Class 12 Notes
- Python Revision tour – 1 Class 12 MCQs
- Python Revision tour – 2 Class 12 MCQs
- Working with Functions Class 12 MCQs
- Using Python Libraries Class 12 MCQs
- File Handling Class 12 MCQs
- Recursion Class 12 MCQs
- Data Visualization using Pyplot Class 12 MCQs
- Data Structure Class 12 MCQs
- Computer Network Class 12 MCQs
- More on MySQL Class 12 MCQs
Computer Science Class 12 Questions and Answers
- Python Revision tour – 1 Class 12 Questions and Answers
- Working with Functions Class 12 Questions and Answers
- Using Python Libraries Class 12 Questions and Answers
- File Handling Class 12 Questions and Answers
- Recursion Class 12 Questions and Answers
- Idea of Algorithmic Efficiency Class 12 Questions and Answers
- Data Structure Class 12 Questions and Answers
- More on MySQL Class 12 Questions and Answers
- Computer Network Class 12 Questions and Answers
- More on MySQL Class 12 Questions and Answers
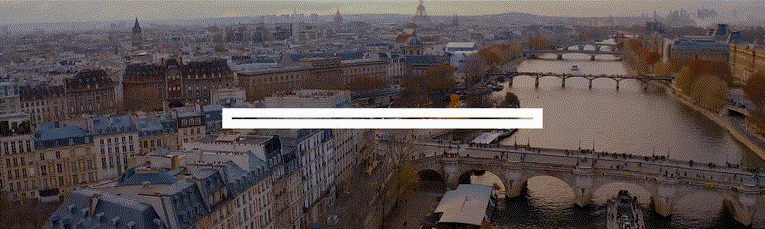
IMAGES
VIDEO
COMMENTS
150+ MCQ Revision Tour of python class 12 Unit -1: Computational Thinking and Programming-2 Topic: Revision of python topics covered in class XI. 1. Which of the following is a valid identifier: a) 9type b) _type c) Same-type . d) True. Show Answer
Python Revision Tour Class 12 Topic Wise MCQ. 1. 140+ MCQ on Introduction to Python. 2. 120 MCQ on String in Python. 3. 100+ MCQ on List in Python. 4. 50+ MCQ on Tuple in Python. 5. 100+ MCQ on Flow of Control in Python. 6. 60+ MCQ on Dictionary in Python.
Class 12 Computer Science 083 Python Revision Tour Set 1 Multiple Choice Questions [MCQs] 1. Who is the founder (designed by) of Python? a) Charles Babbage b) Guido Van Rossum c) Guido Gosling d) James Gosling 2. Python development is started in which year? a) 1990 b) 1991 c) 1992 d) 1989 3. Python is […]
Get answers to all exercises of Chapter 1: Python Revision Tour Sumita Arora Computer Science with Python CBSE Class 12 book. Clear your computer doubts instantly & get more marks in computers exam easily. Master the concepts with our detailed explanations & solutions.
Python revision tour class 12 mcq 2021 pdf download. Core basics. code. str (string, a collection of characters). type () returns the type of its argument. floats. (nor the power-of-ten exponent indicator e). pair of double or single quotes. Quotation marks.
Python Revision Tour Class 12 MCQ. 20/12/202226/11/2022 by CBSEskilleducation. Teachers and Examiners ( CBSESkillEduction) collaborated to create the Python Revision Tour Class 12 MCQ. All the important Information are taken from the NCERT Textbook Computer Science (083) class 12. Contents show.
Python Class 12 | Chapter 1 | Important MCQ's | Python Revision Tour - 1Telegram Group: https://t.me/codeituplearnersTelegram Channel: https://t.me/i_t_s_a_n...
This video is for all class 12 CBSE students, watch the complete python revision tour for class 12.Follow and understand the python concepts with SOLVED impo...
Python Revision Tour (LIVE) | MCQs for Term 1 | Class 12 Computer ScienceMCQs of class 12 computer science, term 1 mcqs for class 12 computer scienceJoin thi...
Class 12 Computer Science 083 Python Revision Tour Set 2 Multiple Choice Questions (MCQs) 21. Is Python case sensitive when dealing with identifiers? a) Yes b) No c) Machine dependent d) None of these 22. Which of the following is an invalid variable? a) name_of_student b) 2_name_of_student c) student_name_2 d) None of these 23. Which […]
Get answers to all exercises of Chapter 2: Python Revision Tour II Sumita Arora Computer Science with Python CBSE Class 12 book. Clear your computer doubts instantly & get more marks in computers exam easily. Master the concepts with our detailed explanations & solutions.
Special characters (#, @, $,%,!) should be avoided in identifiers. Python Revision Tour 1 Class 12 Questions with Answers. 6. What is Variable? Answer -A variable is a location in memory where a value is stored in a computer language. In Python, a variable is formed when a value is put into it.
in Python for Class 12. Multiple Choice Questions: (20 x 1=20) 1. What will be the output of the following Python code? from math import factorial. print (math.factorial (5)) (a) 120. (b) Nothing is printed. (c) Error, method factorial doesn't exist in math module.
05/11/2022 by CBSEskilleducation. Teachers and Examiners ( CBSESkillEduction) collaborated to create the Python Revision Tour 1 Class 12 MCQ. All the important Information are taken from the NCERT Textbook Computer Science (083) class 12. Contents show.
How to execute or run python program. Open python IDLE. Click 'File' and select 'New' to open Script mode. Type source code and save it. Click on 'Run' menu and select 'Run Module'. Python Keywords. These are predefined words which a specific meaning to Python Interpreter. These are reserve keywords.
EDIT: I just noticed a pattern, the answers in itself aren't wrong..its just that, the numbering is wrong. For eg- In Q36. the answer is b) list, but the solution provided is d), this d) part is the answer of Q35..similarly in Q37. the answer is a) part, but the solution provided is D), this d) is the answer of previous question..in simple words answer of Q9 was not present but it got shifted ...
Python Revision Tour - I. Python Revision Tour - II. Working with function. Using Python Libraries. Recursion. Data File Handling: Working with Text files. Data File Handling: Working with Binary files. Data File Handling: Working with CSV files. Data Structure: Searching and Sorting.
chapter - 1 -Python revision Tour -1(One mark, MCQ questions) (1) - Free download as PDF File (.pdf), Text File (.txt) or read online for free. chapter - 1 -Python revision Tour -1(One mark, MCQ questions) (1)
15 MCQs File handling computer science class 12 | board questions computer science class 12 Join this channel to get access to the perks:https://www.youtube....
Python Revision Tour - II quiz for 12th grade students. Find other quizzes for Computers and more on Quizizz for free!
05/11/2022 by CBSEskilleducation. Teachers and Examiners ( CBSESkillEduction) collaborated to create the Python Revision Tour Class 12 Notes. All the important Information are taken from the NCERT Textbook Computer Science (083) class 12. Contents show.
MCQ CHAPTER - REVISION TOUR CLASS TEST - I Class XII Sub : CS (083) Time: 40 Min Max Marks: 20 1. Which of these in not a core data type? a) Lists b) Dictionary c) Tuples d) Class 2. Given a function that does not return any value, What value is thrown by default when executed in shell. a) int b) bool c) void
MCQ for Class 12 Computer Science Python. Python Revision tour - 1 Class 12 MCQs. Python Revision tour - 2 Class 12 MCQs. Working with Functions Class 12 MCQs. Using Python Libraries Class 12 MCQs. File Handling Class 12 MCQs. Recursion Class 12 MCQs. Data Visualization using Pyplot Class 12 MCQs. Data Structure Class 12 MCQs.